Bio
This page serves as a portfolio containing my programming projects. I was first exposed to programming when I was around 10 years old, when I taught myself the basics of JavaScript by messing around with my browser's javascript console. I wanted to learn javascript because I was exposed to it through cookie clicker, when I used the javascript console to edit the prices of items and change internal game variables. I then started creating my own video games when I was around 10-11 years old in a game engine called "Game Maker Studio 1.4". Through developing games I discovered that I have a passion for programming. Eventually, instead of just making games, I began writing my own software and scripts. Over the years I have been building up my programming ability. The projects on this page are relatively newer projects of mine, mostly programmed in C.
Programming Philosophy
I mostly program in C89 because I find it to be a simple and beautiful language. One thing that I appreciate about C89 is how the compiler forces you to put the variable declarations at the top of functions, which encourages structured thinking. I also love that C is a procedural language. While I can adapt to object-oriented or functional paradigms, I personally prefer the procedural style. In C, defining types is simple - there's no need for objects or classes. I enjoy the simplicity of having functions manipulate data structures directly, without the overhead of complex class hierarchies.
C is simple because it is explicit and avoids the "magic" often found in OOP languages. For example, polymorphism in C can be achieved using unions and enums. All possible types are explicitly defined in an enum, and the active type is determined by the programmer. This makes the code more readable, understandable, and easier to debug. I also prefer static, compiled languages like C over scripting languages because they reduce the likelihood of runtime errors caused by dynamic typing and other things. For me, a program's behavior should always be visible and predicitable just by reading its source code.
For these reasons I also value the skill of writing clear and simple code. I find that with other languages there is often a culture of writing "clever code". What I mean by this is cases where people will sacrifice legibility in order to write something they think makes them look clever. I see this a lot in python code where people often try to fit their code into unreadable "one-liners". For example here is a case of some clever code. It must be better because it fits in one line right?
result = [x**2 for x in range(10) if x % 2 == 0]
Something like the above code snippet should never be valued over clean and concise code. Here is the same code but unwrapped into something that is easy to read (both code snippets produce the same exact result). The logic is separated into multiple easy to read steps:
result = []
for x in range(10):
if x % 2 == 0:
result.append(x**2)
Software Applications & More
Portamento (2024)
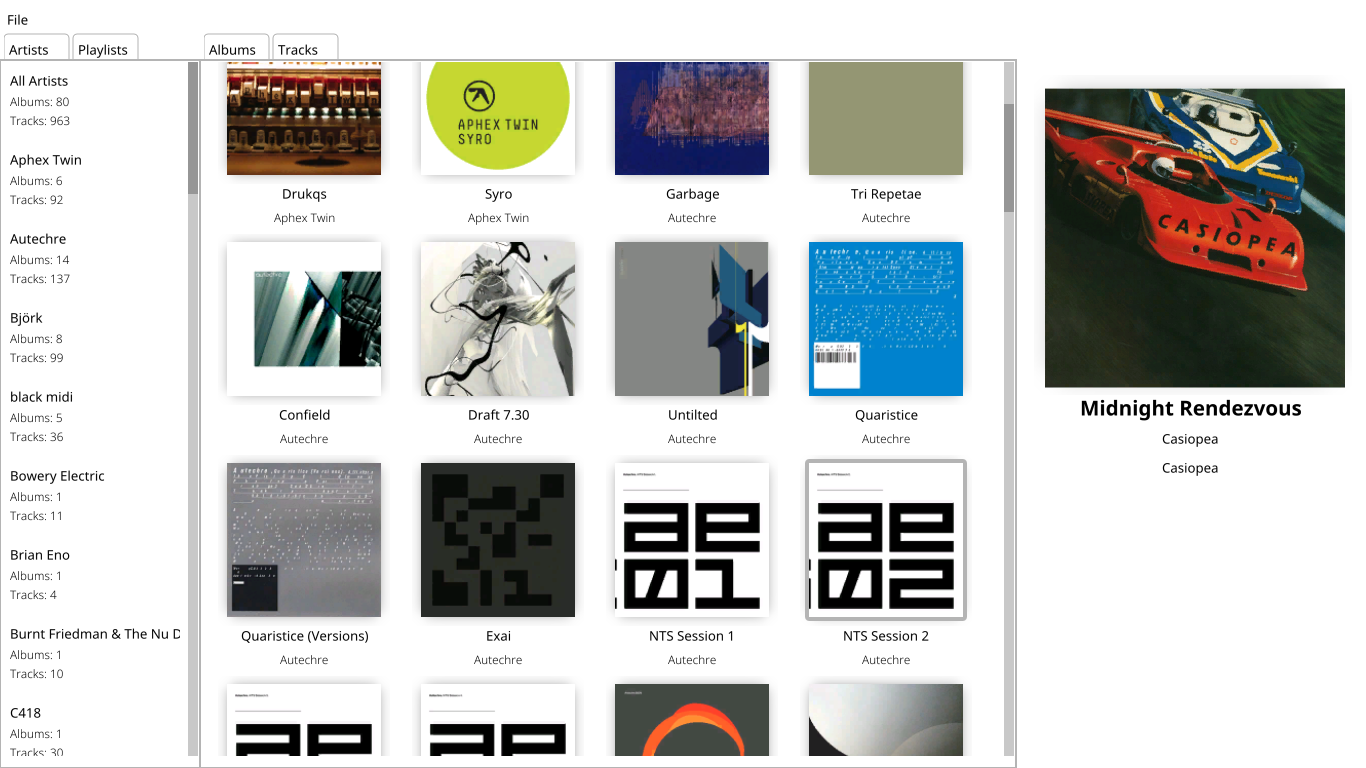
A music player for linux written in C89, using OpenGL and Nuklear.h for rendering. It features fast metadata loading and efficient GPU memory management for album art.
Features- GUI rendered with OpenGL.
- Multithreaded metadata processing and event handling. Album artwork is also processed on its own thread.
- Album art stored in GPU memory with DXT compression to minimize RAM and VRAM usage.
- Metadata stored in an SQL database for instant loading.
- Scales efficiently to large music libraries.
Experimental Game Jams (2022)
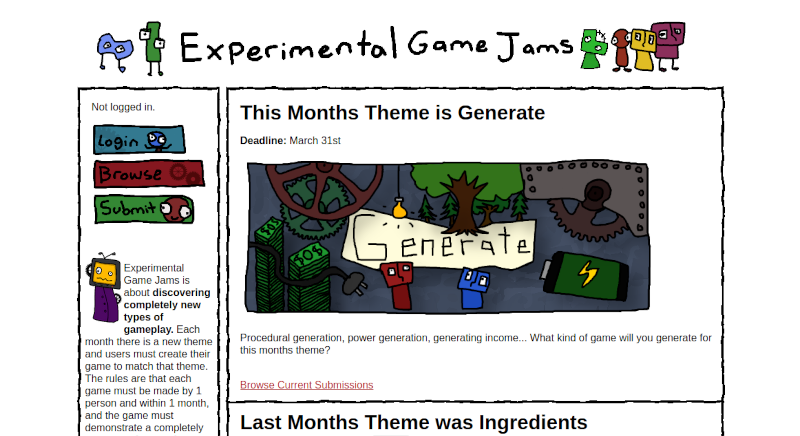
Experimental Game Jams is a website where users can upload their own experimental video games. Each month there is a new theme and users must create their game to match that theme. The rules are that each game must be made by 1 person and within 1 month, and the game must demonstrate a completely new type of gameplay never seen before.
Features- User accounts, profiles, star-rating, comments
- Email confirmation to prevent accounts being created en-masse.
- The ability to upload games for multiple platforms.
- Browse page where you can sort results by different metrics.
- User accounts can have bios and profile pictures.
NoteWM (2023)
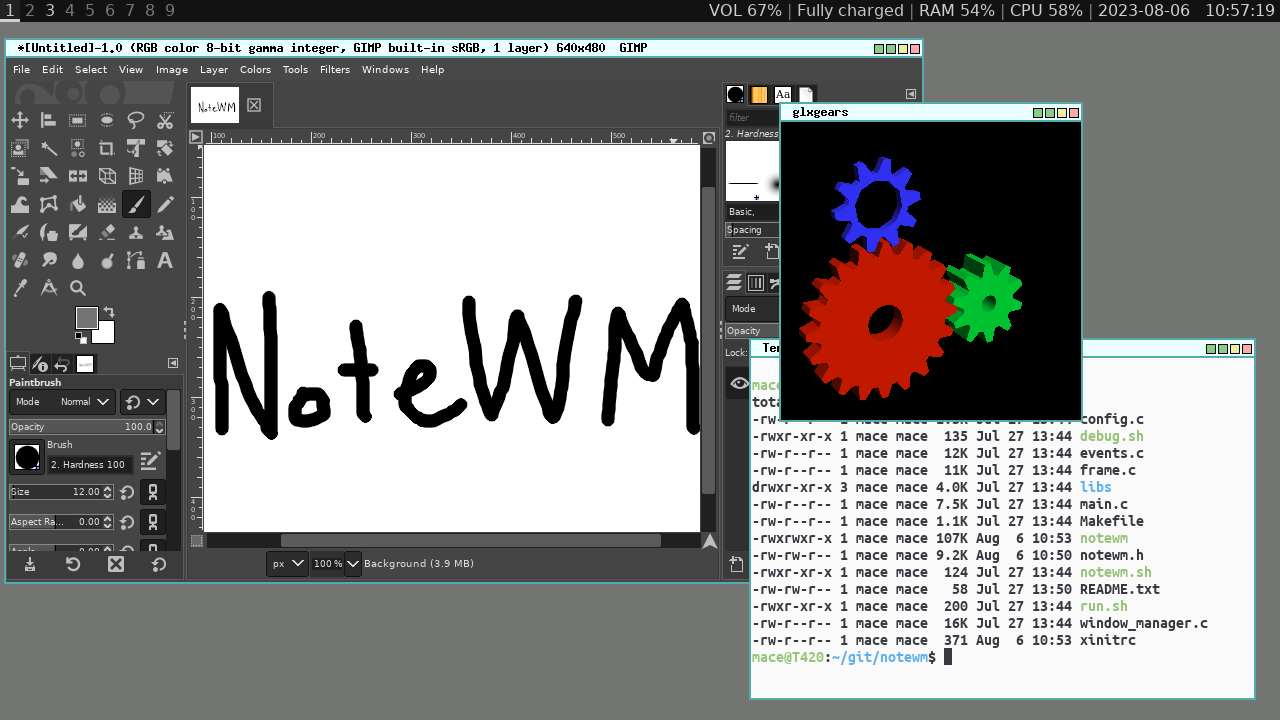
NoteWM is a simple X11 floating reparenting window manager. It is written in C89 and uses the Xlib library. I created this project to learn more about the Xlib library and how window managers actually work. It was a great learning experience because Xlib and window managers (especially reparenting window managers) aren't well documented online. A lot of learning took place by looking at the source code of the window managers I use on a daily basis to figure out how they worked. It's still not entirely finished, but one day I hope to return to it and implement the features I want so that I can use it as my main window manager.
Features- Windows can be freely moved around, resized, and created on different workspaces.
- Implements some extended window manager hints so that applications like polybar or other status bars can function.
- Custom colors can be applied.
- Manages window decorations by reparenting client windows (these are the buttons, titles, and frames on each application window).
Illuscribe (2023)
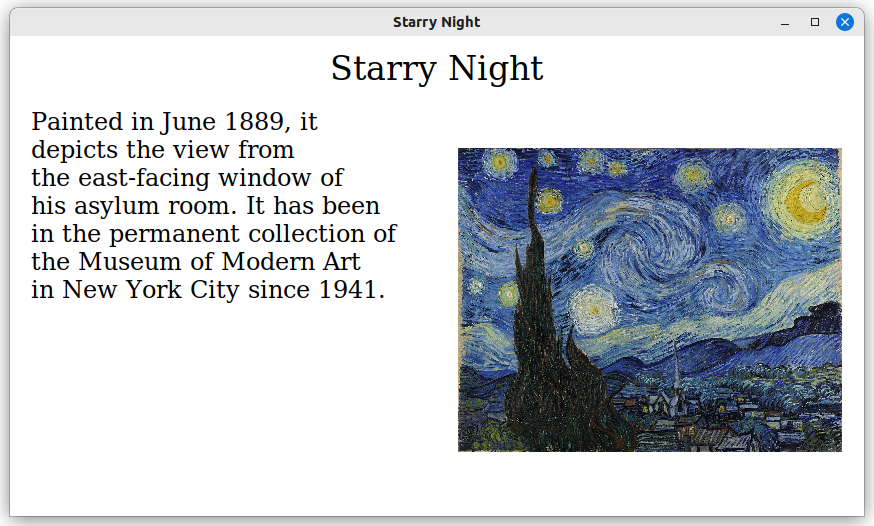
Illuscribe is an application that allows you to present slideshows from plaintext files. It is written in C89 and uses the Xlib library as well as stb_image.h for loading images. It parses plaintext files and renders them as presentations. Below is an example of the markup language I created for making slideshows:
template: "my-template"
box: "title-box", stack-vertical, align-center
box: "content-box", stack-horizontal, align-left
end
slide: "slide1"
uses: "my-template"
define: "title-box"
text: title, "Hello World!"
end
define: "content-box"
text: normal, "This is some text."
image: "yourimage.png"
end
end
Features:
- A markup-style language to create slideshows and templates in plaintext.
- Dynamically handing layouts of slides.
- Different layout options, font sizes, and the ability to insert images into your slides.
- Templates for re-using the same layout across multiple slides.
- Slides can be inserted into other slides, so that you can append text to an existing slide. For example: a bullet point appearing in a list one at a time.
txt2web (2023)
-----
title: Your Blog Title
date: May 11 2023
description: This is the HTML meta description
tags: these, are, comma, seperated, meta, keywords
style: custom.css
-----
# Markdown style headings
## heading size depends on number of pound signs
Images can be added using an @ sign and a filename:
@/images/image.png
Code blocks can also be written like in markdown:
```
int main(void)
{
printf("This is a code block!\n");
return 0;
}
```
A simple static site generator for creating a blog. This C program turns a directory of plaintext files into a full html website.
Features- Custom markdown-style syntax
- Parses plaintext files and converts them to HTML. The HTML pages are then organized by date on the homepage.
- Each blog post text file has metadata for the title, date, meta description, and meta tags. The title and date appear on the homepage.
tuxdoc (2023)
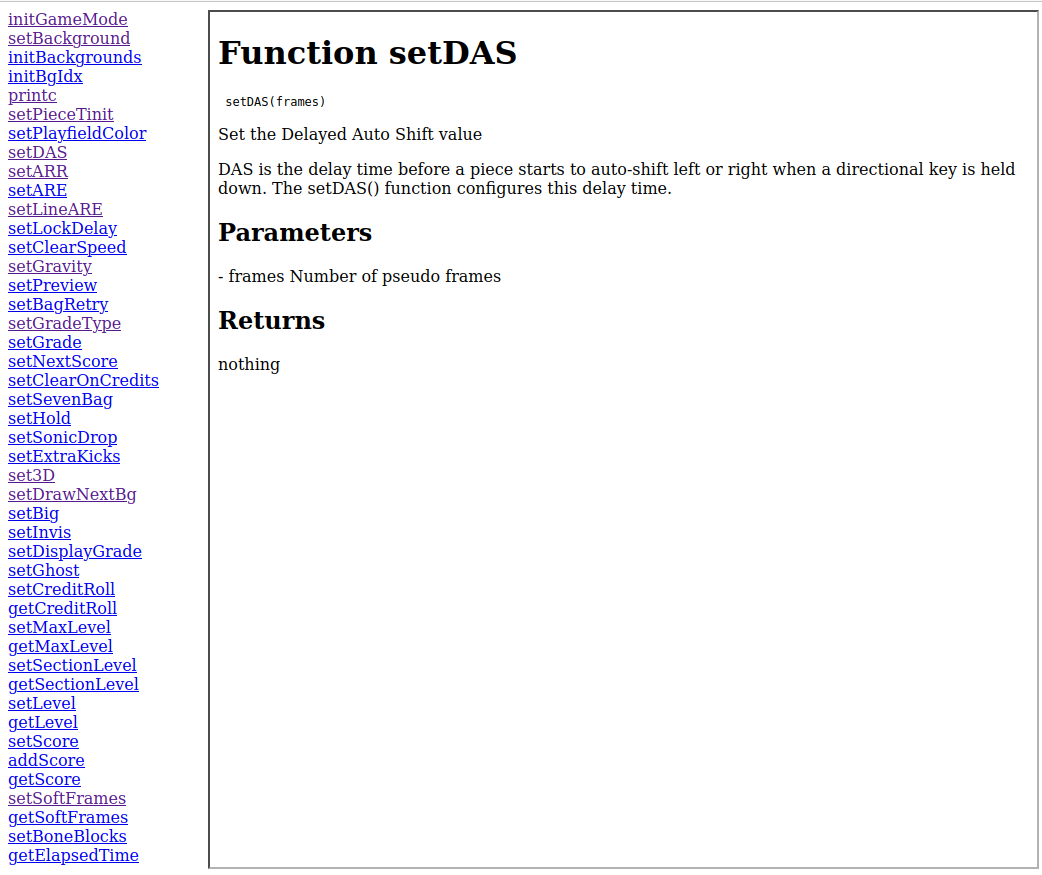
Generate lua documentation from C files. Created specifically for my game tuxmino. This program will look in a c file for docstrings formatted like the code snippet below and convert them into an HTML documentation page:
/*
* lua function
* @brief a brief description
* A full summary. Can be multiple lines.
*
* @param parameter_name Description of parameter
* @returns return_type Description of return type
* @usage functionPrototype(parameter_name)
*/
boids2d-rs (2023)
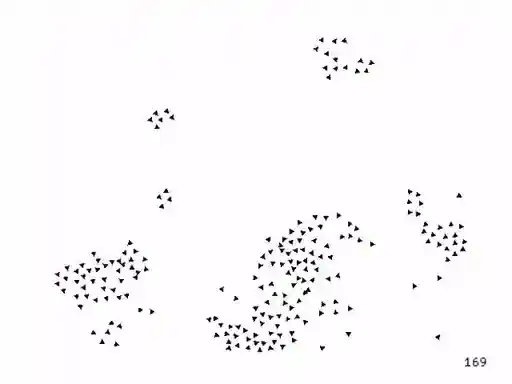
Boids flocking simulation written in Rust. Uses the macroquad library for rendering. This was my first time using the rust programming language. This implementation is using the naive O(n^2) implementation. I might implement a more optimized version of the simulation in the future, possibly in C (and maybe in 3d instead of 2d).
Features- The ability to place obstacles, which the boids will avoid.
- The ability to place "food" which the boids will flock towards.
Karplus Strong String Synthesizer (2023)
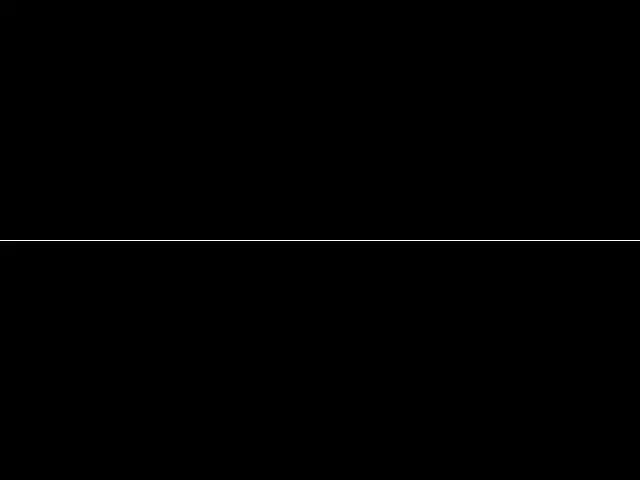
Implementation of the Karplus Strong algorithm for synthesizing the sound of a plucked string. This Wikipedia article and this page from a columbia.edu textbook helped me understand how the algorithm works. This was a good little project for learning about how audio buffers work.
Features- Waveform visualization using SDL2 for rendering.
- Real-time audio playback with the ability to play multiple notes at once.
Games
Tuxmino (2022)
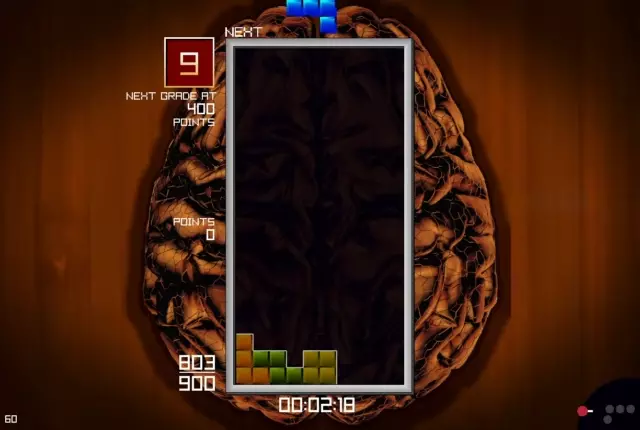
Tuxmino is a highly moddable free open source falling block puzzle game. It is written in C using the raylib game library. The goal of this project was to create a highly polished open source TGM clone (TGM is a japanese arcade version of Tetris, known for being extremely challenging). I was unhappy with other open source games similar to this, so I made my own. It also has support for making your own custom game modes in LUA. The main game mode is an exact (as exact as I could get it) replica of the first TGM game. Everything from the rotation mechanisms, speed timings, frame timings, kicks, DAS, lock delay, and more are exactly the same as the real arcade game. A lot of research went in making the game as polished and true to the original as I could. I even consulted people who had the grand-master ranking in the real arcade games to get their feedback on my version.
Features:- An entire LUA api for making your own custom game modes (without having to re-compile the game). Simply put a lua script in the gamemodes/ folder and the gamemode will appear in-game.
- All the necessary speed timings (DAS, Lock delay, appearance delay, etc), animations, and more to make the experience as polished and professional as possible.
- Multiple rotation rulesets, one for replicating older arcade games, and another for replicating the newer rotation mechanisms found in modern guideline tetris games.
- Ability to map your own keybinds.
- Two websites: tuxmino.org for info on contributing, tutorials for making your own gamemodes, and more. docs.tuxmino.org for the lua api documentation (which is auto generated from source code comments using my tool tuxdoc.
Gupta (2023)
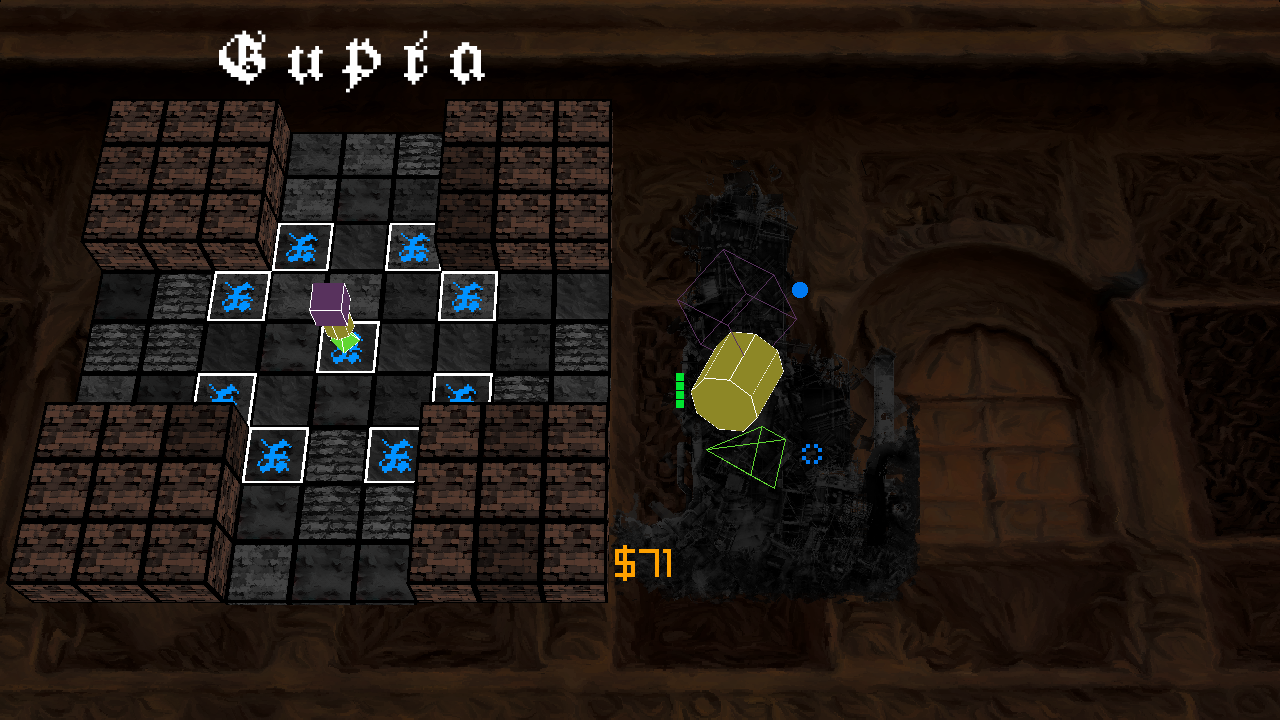
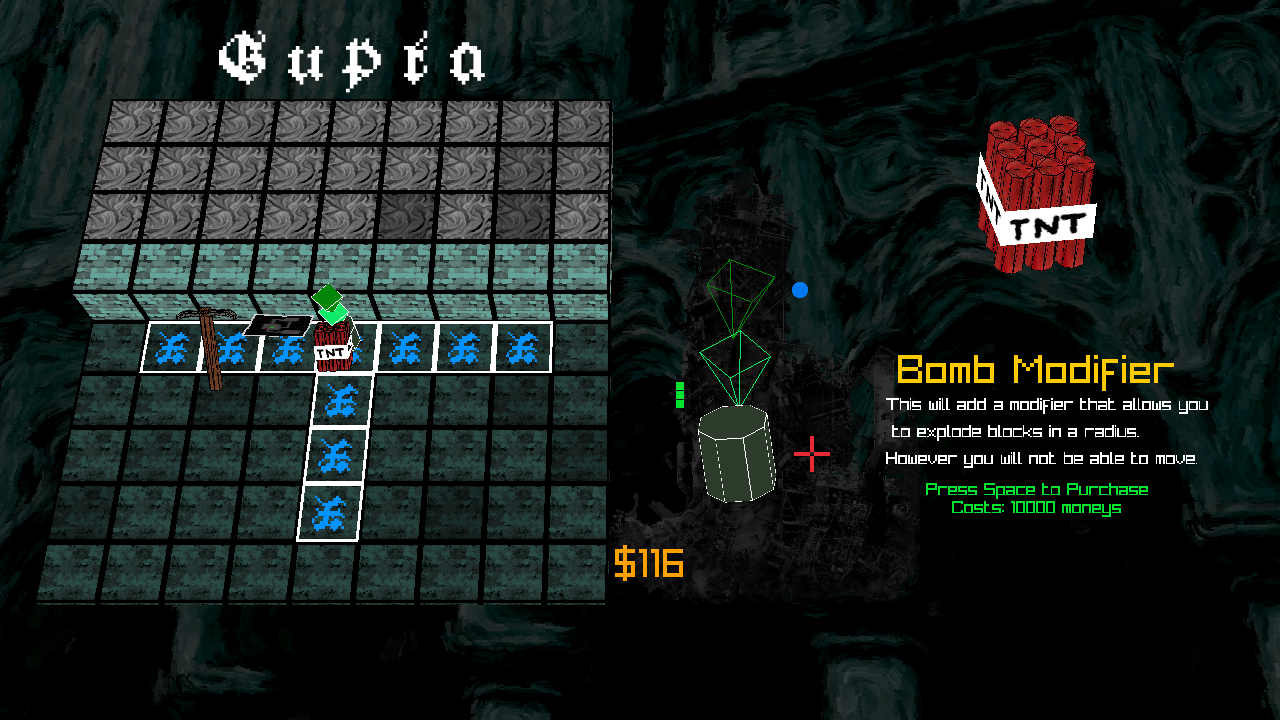
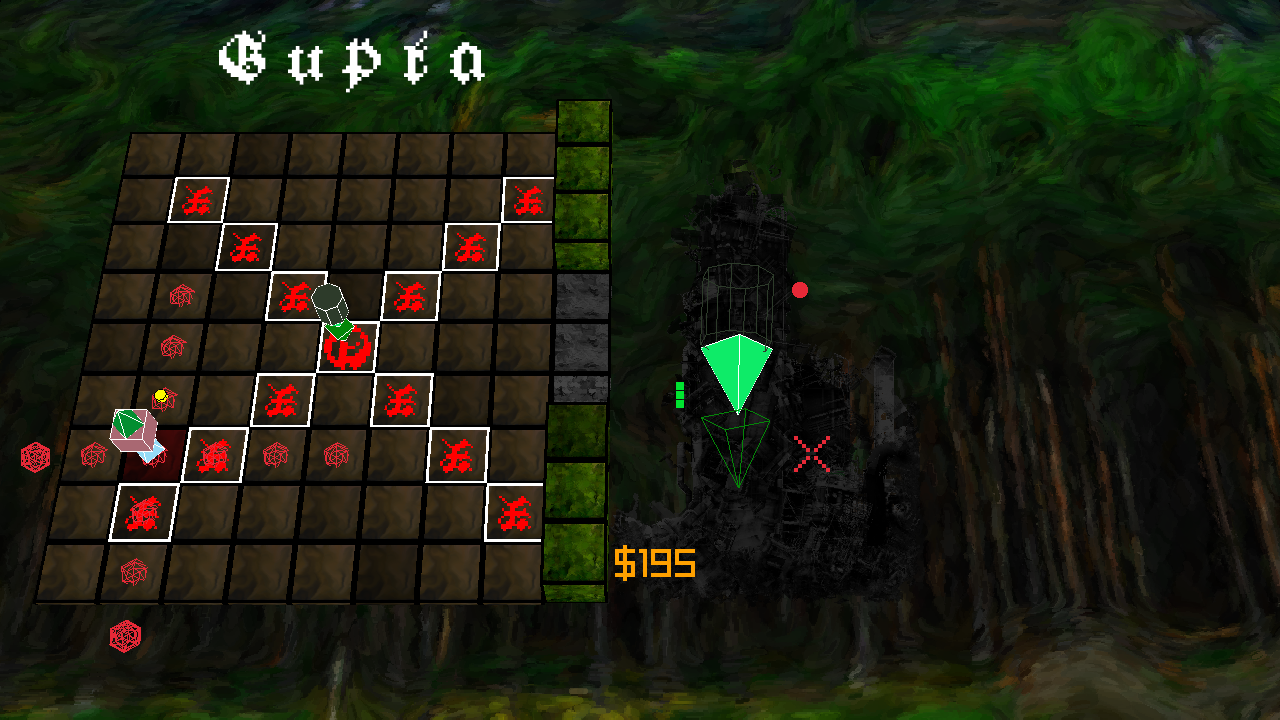
Gupta is a rogue-like with chess based movement. You can combine different segments of your 'chess piece' to alter your move pattern. This game was created in 2 weeks for my experimental game jams website. You can view the submission here. The theme for this jam was 'grid'. This game is written in C and uses the raylib game library.
Features- Random dungeon generation with mutliple different biomes.
- Chess based movement, using the bresenham line algorithm to check for valid movement.
- Enemies with basic AI that have their own chess-like move patterns. Enemies ocassionally make mistakes just like a player would, which makes it possible to defeat enemies with any type of move pattern.
- The ability to pick up chess piece parts from defeated enemies. You can then combine multiple chess pieces to alter your move pattern.
- Different modifiers allowing you to jump over walls (knight-like movement on any move-pattern), mine walls, or even explode walls in a certain radius.
- Shop which contains different upgrades, one of which is a minimap.
Cat's Cradle (2023)
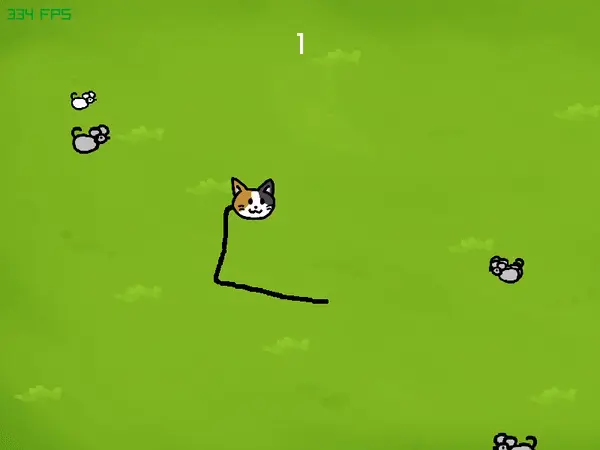
Another game I made for experimental game jams. In this game you use your tail to draw circles around mice in order to capture them. I made this game in about one day. It is written in C and uses the raylib game library.
Features- The cat's movement leaves a trail (representing its tail), which players use to encircle mice.
- The tail is drawn using interpolated points between vectors to smoothly render the tail.
- The game checks for loops using a line-segment intersection algorithm to check if any tail segments intersect one another.
- The game checks if a mouse is inside a loop by using a point-in-polygon algorithm and ray-casting.
3D Animations
These are animations I've created for my YouTube Channel. They are made in the Godot game engine. I am not experienced with animation, so all the animations and events in these videos are controlled through code (GDScript). I also use custom shaders for rendering different effects. The 3d models are also created by me in a program called Blender.
Writer's Block (Animation) (2024)
111ee8 (Music Video) (2024)
This video contains strange shapes that are synced to the music (midi events). The shapes were generated using a simple python script I wrote. Each shape is created by generating random points in a 3d space and then connecting faces between the points.
import numpy as np
import trimesh
import random
def rand_mesh():
vertices = np.random.rand(random.randint(20, 100), 3)
faces = []
for _ in range(len(vertices) - 2):
face = tuple(np.sort(random.sample(range(len(vertices)), 3)))
if face not in faces:
faces.append(face)
faces = np.array(faces)
return trimesh.Trimesh(vertices=vertices, faces=faces)
def main():
for i in range(50):
mesh = rand_mesh()
mesh.export(f'random_mesh_{i}.obj')
if __name__ == "__main__":
main()